Laravel and Visual Studio Code
If you want to develop on Laravel framework, and your favorite IDE is Visual Studio Code, this post is for you.
- Visual Studio Code allows you to define tasks to run anything.
- Laravel allows you to run a ‘live’ version of your project running a command.
1 + 1
The command Laravel offers to run a live instance is next one:
php artisan serve
This comand will start a development server in 127.0.0.1:8000
This command accepts multiple arguments, one of the most useful is env. It provides the possibility of start a live instance of a custom environment.
But wait… What is a Laravel environment?
A Laravel environment is a file called .env.XXX which contains all the especifications of:
- Components configurations:
- Database
- Queues
- SMTP
- AWS Storage
- Notifications
- Application details like name, owner…
- And any environment variable we define.
Creating a Laravel environment
The easiest is to copy the example environment to something usable:
cp .env.example .env.local
Edit .env.local file to use sqlite database (allows you to use in memory volatile database and develop on the project without the need of giving up a MySQL server.)
APP_NAME="Nuytsy NMS" APP_ENV="development" APP_OWNER="The Nutsy Company" APP_KEY= APP_DEBUG=true APP_URL=http://localhost LOG_CHANNEL=stack DB_CONNECTION=sqlite DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=/your/vscode/workspace/folder/database/database.sqlite DB_USERNAME=root DB_PASSWORD=
But.. Wait, what is my APP_KEY? No problem, generate it by running:
php artisan key:generate --env=local
Defining local we are telling Laravel to use our .env.local file
Be careful, Laravel won’t create the database.sqlite file for us, we need to create it. If this file does not exist, then your ‘live’ environment won’t be able to use a database.
# Remember to use your real path touch /your/vscode/workspace/folder/database/database.sqlite
Ok, so now we can launch a ‘local instance’ to see how our project is working.
php artisan serve --env=local
Creating a task in Visual Studio Code
Use Command Palette (Ctrl+Shift+P) and write ‘Task’, you should view following options:

Select configure task, then create task.json file and select the template others

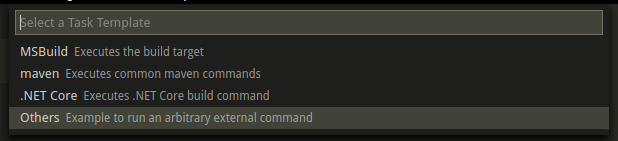
This action will create a task.json file in your .vscode folder with following content:
{ // See https://go.microsoft.com/fwlink/?LinkId=733558 // for the documentation about the tasks.json format "version": "2.0.0", "tasks": [ { "label": "echo", "type": "shell", "command": "echo Hello" } ] }
Simply, we’ll customize this file to register our desired action Run laravel
{ // See https://go.microsoft.com/fwlink/?LinkId=733558 // for the documentation about the tasks.json format "version": "2.0.0", "tasks": [ { "type": "shell", "label": "Run laravel", "command": "php", "args": ["artisan", "serve", "--env=local"], "options": { "cwd": "${workspaceFolder}/" }, "group": "test" } ] }
So, now we can run a new task called Run laravel:


If we activate this a new terminal will appear, with our command running:
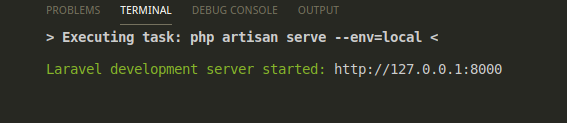
We could now navigate to http://localhost:8000 and start viewing how our project is working with a valid database connection:
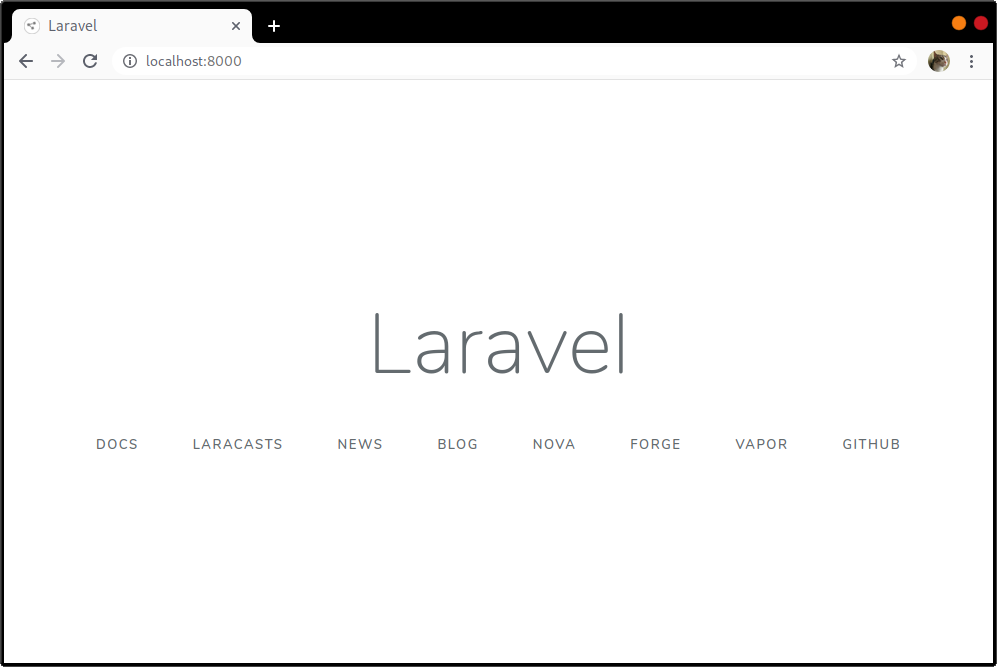
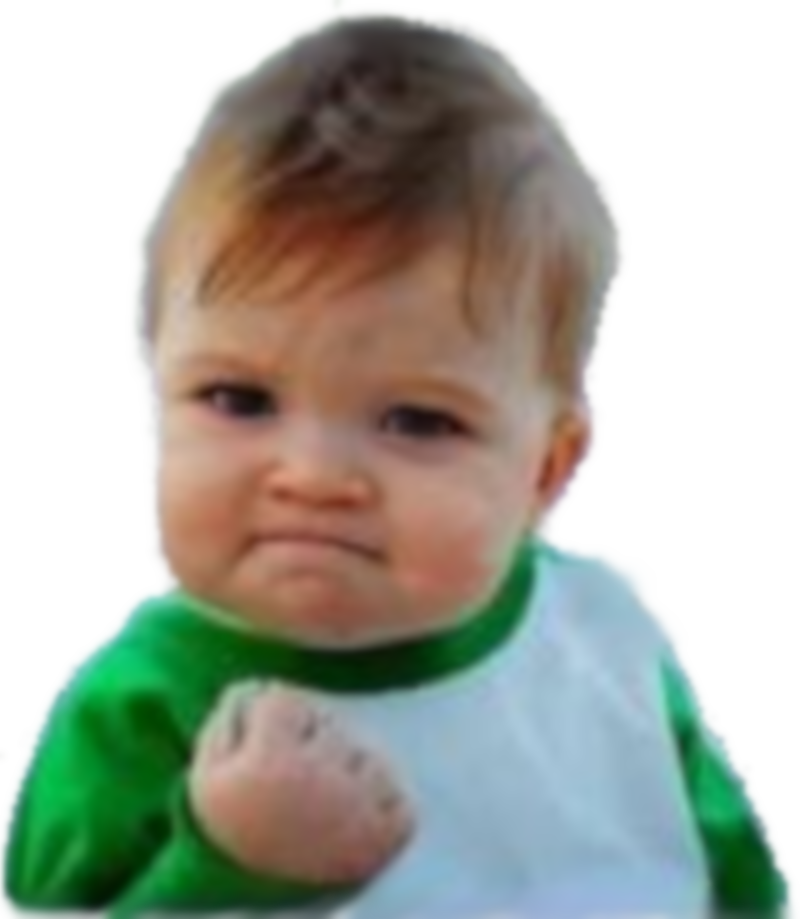